Solidity Language Example:A Guide to Solidity Programming in Smart Contracts
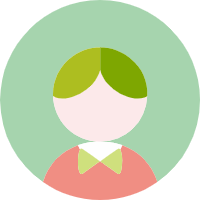
Solidity is a programming language designed specifically for creating smart contracts for the Ethereum blockchain. It is a flexible and powerful language that allows developers to create secure, transparent, and decentralized applications. In this article, we will provide an overview of the Solidity language and provide an example to help you get started.
Solidity Overview
Solidity is a dynamic, open-source, and object-oriented programming language. It is designed to be easy to learn and use, while still providing the flexibility necessary to create complex smart contract applications. Solidity supports several features, including:
1. Arrays and strings
2. Conditional statements (if, else if, else)
3. Loops (for, while)
4. Functions
5. Classes and structures
6. Event handling
7. Modules and interfaces
8. Memory and stack management
Solidity Example: A Simple Smart Contract
Now that we have reviewed the basics of Solidity, let's create a simple smart contract example. The following code demonstrates a smart contract that allows users to add two numbers and display the result:
```solidity
pragma solidity ^0.8;
contract SimpleCalculator {
struct Data {
uint256 result;
}
mapping(address => Data) public users;
function setValue(uint256 input) public returns (uint256) {
users[msg.sender].result = input;
return users[msg.sender].result;
}
function getResult() public view returns (uint256) {
return users[msg.sender].result;
}
}
```
In this example, we defined a `Data` structure to store the result of the calculation. We also created a mapping to store the user's input and the result. The `setValue` function allows users to set their input, and the `getResult` function allows users to view their result.
Running the Smart Contract
To run the smart contract, you need to install Ethereum's Remix IDE or use a web3 browser such as MetaMask. Once you have set up your environment, follow these steps to deploy the smart contract to the Ethereum network:
1. Open Remix IDE or your preferred Ethereum client.
2. Enter the Solidity code from the example into the code editor.
3. Press the "Deploy" button and select the appropriate network (e.g., Mainnet, Ropsten, Ropay).
4. Confirm the deployment by typing in the sender's address and pressing the "Deploy" button again.
5. The smart contract will be deployed to the selected network, and you can view its address in the output window.
In this article, we provided an overview of the Solidity programming language and demonstrated a simple smart contract example. Solidity is a powerful and versatile language that allows developers to create secure and efficient smart contracts for the Ethereum blockchain. By understanding the basics of Solidity and practicing with simple examples, you will be well on your way to creating your own smart contract applications.