Create Smart Contract Using Solidity Programming:A Guide to Developing a Smart Contract on the Ethereum Network
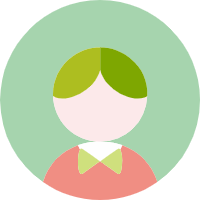
Smart contracts are self-executing contracts with terms and conditions written in code. They are designed to automatically execute the terms of a contract without the need for third-party intervention. The Ethereum network, one of the most popular smart contract platforms, allows developers to create, deploy, and manage contract-based applications using its native token, ether (ETH). This article provides a step-by-step guide on how to create a smart contract using Solidity programming, the primary language for developing smart contracts on the Ethereum network.
1. Understanding the Basics of Smart Contracts
Smart contracts are written in a high-level programming language called Solidity. They are compiled into a bytecode, which is then deployed to the Ethereum network. Once deployed, the smart contract can interact with the underlying blockchain, enabling it to execute tasks and processes according to its pre-programmed conditions.
2. Installing the Required Tools and Libraries
To develop smart contracts, you need to install the following tools and libraries:
- Node.js: A JavaScript-based runtime environment used for developing and running Solidity contracts.
- Solidity: A high-level programming language designed specifically for creating smart contracts.
- Remix IDE: An online IDE that allows you to write, compile, and deploy Solidity contracts.
3. Creating a Simple Smart Contract
Let's create a simple smart contract that displays the current time and temperature when a user calls a function named "getTimeAndTemperature". The following is the Solidity code for the contract:
```
pragma solidity ^0.8;
contract SimpleSmartContract {
uint256 time;
string temperature;
function getTimeAndTemperature() public view returns (uint256, string memory) {
return (time, temperature);
}
}
```
4. Compiling and Deploying the Smart Contract
Once you have written the smart contract code, compile it using Node.js and the remix IDE. You can find the compiled code in the "build" folder. Next, deploy the compiled smart contract to the Ethereum network using Remix IDE or the "deploy" button in the "build" folder. You will need to provide the network's gas limit and the value of the ether token to deploy the contract.
5. Interacting with the Smart Contract
Once the smart contract is deployed, you can interact with it using any Ethereum client, such as MetaMask or Web3.js. For example, you can call the "getTimeAndTemperature" function using a transaction sent from your browser or a separate node client. The response will include the current time and temperature.
6. Advanced Features and Best Practices
As your smart contract grows more complex, it's important to consider advanced features and best practices. Some key points to keep in mind include:
- Encoding data: Encoding data within the smart contract can help reduce storage costs and ensure that the contract remains compact.
- Access control: Implementing access control can help secure the smart contract and prevent unauthorized access.
- Recoverable contracts: Using recoverable contracts can help prevent vulnerabilities such as reentrancy attacks and balance erosion.
Developing smart contracts using Solidity and the Ethereum network is a straightforward process. By following this guide, you can create sophisticated smart contract-based applications that can automate tasks and processes, reducing the need for third-party intervention. As you progress in your smart contract development, be sure to explore advanced features and best practices to ensure the security and efficiency of your contracts.