Portfolio Volatility Formula Python: A Guide to Implementing Portfolio Volatility Formulas with Python
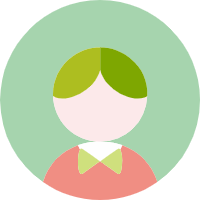
Portfolio volatility is a crucial aspect of investment management, as it affects the overall risk and return of a portfolio. Calculating and understanding portfolio volatility is essential for making informed investment decisions. In this article, we will explore the portfolio volatility formula in Python and provide a guide on how to implement it. We will also discuss the benefits of using Python for portfolio volatility calculations and provide some practical examples.
Portfolio Volatility Formula
The portfolio volatility formula is used to calculate the volatility of a portfolio's return. It is calculated by taking the standard deviation of the returns of each asset in the portfolio and weighting them by their market values. The formula for portfolio volatility is:
Portfolio Volatility = sqrt[Σ(Asset Return i * Weight i)^2]
where Asset Return i is the return of the ith asset, Weight i is the weight of the ith asset in the portfolio, and i runs from 1 to n, where n is the number of assets in the portfolio.
Implementing the Portfolio Volatility Formula in Python
Python is a powerful programming language that can be used to implement the portfolio volatility formula. We will use the `numpy` and `pandas` libraries to perform the calculations. First, we need to import the necessary libraries and create a sample portfolio with assets and their weights:
```python
import numpy as np
import pandas as pd
# Create a sample portfolio with asset returns and weights
asset_returns = np.array([0.1, 0.2, 0.3, 0.4, 0.5])
asset_weights = np.array([0.25, 0.25, 0.25, 0.25, 0.25])
# Calculate the portfolio volatility
portfolio_volatility = np.sqrt(np.dot(asset_returns * asset_weights, np.abs(asset_returns * asset_weights) ** 0.5))
```
You can replace the sample asset returns and weights with your own data to calculate the portfolio volatility for your specific portfolio.
Benefits of Using Python for Portfolio Volatility Calculations
1. Code Reusability: The portfolio volatility formula can be implemented in multiple languages, but using Python makes it easier to reuse the code and adjust it for different portfolios and assumptions.
2. Easy Integration with Other Tools: Python can be easily integrated with other financial tools and libraries, such as MATLAB, R, or Excel, to perform more advanced portfolio volatility calculations and analysis.
3. High-Performance Calculations: Python is a highly optimized programming language that can handle large volumes of data and complex calculations quickly and efficiently.
4. Flexibility and Customization: Python allows for flexible and customizable code, making it easy to adapt the portfolio volatility formula to different investment strategies and risk management methods.
In this article, we have explored the portfolio volatility formula and provided a guide on how to implement it in Python. Using Python for portfolio volatility calculations offers numerous benefits, such as code reusability, easy integration with other tools, high-performance calculations, and flexibility and customization. By understanding and implementing the portfolio volatility formula in Python, you can make more informed investment decisions and better manage the risk of your portfolio.